What is a virtual environment?
Virtual environments are useful tools in Python development that allow you to isolate package installations related to a specific project from the main system’s Python installation. This means you can have separate environments with different package dependencies for different projects.
Creating a virtual environment is easy using the venv module in the Python standard library. The basic steps are as follows:
- Create the virtual environment by running the following command:
python3 -m venv venv
This will create a venv folder that contains the isolated environment.
- Activate the virtual environment.
On Unix/Linux systems, run the following command:
source venv/bin/activate
On Windows, run the following command:
venv\Scripts\activate
Your command prompt will now show the virtual environment name enclosed in parentheses. Any package installations will now be isolated in this environment.
- You can install any packages needed for your project using pip:
pip install package-name
- When you are done, you can deactivate the environment and switch back to the global Python install with the following command:
deactivate
Virtual environments make it easy to try out different package combinations for your projects without interfering with the global Python install or other virtual environments.
In order to have a complete view of virtual environments in Python, please check the Real Python website (https://realpython.com/python-virtual-environments-a-primer/#use-third-party-tools):
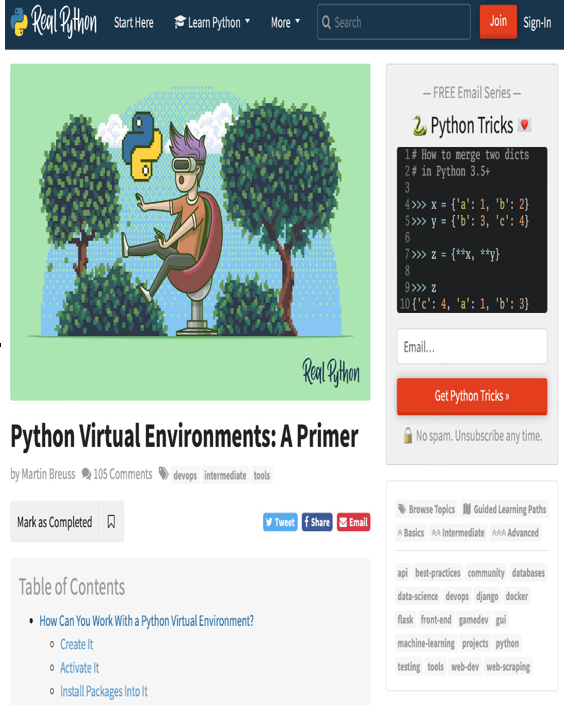
Figure 2.10: Python virtual environments on the Real Python website
Apart from the built-in venv module, there are several third-party tools such as virtualenv and pipenv for managing virtual environments in Python. The following bullets list the various benefits of using these third-party tools:
- Easier to use: The virtualenv and pipenv tools come with some additional convenience features that make them easier to use than the venv module. For example, pipenv automatically creates a Pipfile to track package dependencies and versions.
- More features: These tools offer some additional features beyond just isolating package installations: for example, pipenv manages both the environment and package dependencies, making your setup fully reproducible.
- More flexibility: Some projects prefer these tools over venv as pipenv has features to help manage development dependencies separately from production dependencies.
- Better compatibility: These tools work on all major platforms and have been around for longer, so they tend to have fewer quirks and compatibility issues. The venv module is a newer addition to the Python standard library.
- Independent of Python version: Since virtualenv and pipenv are third-party tools, they can work with different Python versions, unlike venv, which is tied to a specific Python installation.
So, in summary, while the venv module is part of the Python standard library, third-party tools such as poetry and pipenv offer more features, flexibility, and compatibility for managing your virtual environments. But for simple needs, the venv module works well and has the benefit of being built into Python itself.